In the dynamic landscape of software development, where adaptability is a fundamental virtue, the Adapter Design Pattern emerges as a pivotal solution. As applications evolve and technologies progress, the necessity to integrate new components or ensure compatibility between existing ones becomes evident. Design patterns, serving as elegant solutions to common problems, play a crucial role in this context. The Adapter Design Pattern, in particular, stands out as an invaluable tool for achieving seamless system interoperability.
Table of Contents
What?
Adapter pattern is a structural design pattern that allows incompatible interfaces to work together by providing a wrapper that converts one interface into another.
Why?
The Adapter Design Pattern is a powerful tool in software development for several reasons, addressing challenges that arise due to changes in requirements, integration of legacy code, and the need for system flexibility. Here are some key reasons why the Adapter Pattern is essential:
- Integration of Legacy Code: In many projects, there’s existing code that may be considered legacy but still holds significant business logic. This code might have been developed with older standards or technologies and may not align with modern interfaces. Adapters enable the integration of this legacy code into new systems without requiring extensive rewrites or modifications.
- Third-Party Library Integration: When incorporating third-party libraries or components into a system, there’s often a chance that their interfaces don’t match the expectations of the existing codebase. Adapters act as intermediaries, allowing the new component to seamlessly integrate with the rest of the system.
- Interoperability: In a heterogeneous system environment, components developed by different teams or organizations may have incompatible interfaces. Adapters act as translators, enabling these components to work together cohesively. This promotes interoperability and collaboration in complex software ecosystems.
- Code Reusability: Adapters encapsulate the logic required for interface conversion, making it reusable across different parts of the system. This not only reduces redundancy but also contributes to a more maintainable and modular codebase.
- Minimal Impact on Existing Code: The Adapter Pattern allows for the introduction of new functionalities without affecting the existing codebase. This is particularly beneficial in situations where modifying existing code might be risky or time-consuming.
- Smooth Migration and Upgrades: When migrating from one technology stack to another or upgrading existing components, the Adapter Pattern can facilitate a smoother transition. It allows for the phased integration of new components, reducing the impact on the overall system.
The Adapter Design Pattern is a crucial asset in a developer’s toolkit, offering a clean and efficient way to handle interface mismatches and promote system adaptability. It helps bridge the gap between different components, fostering a cohesive and resilient software architecture.
Code
we have a legacy XML logger, which is now replaced by a JSON logger. The adapter enables us to use both.
# New Logger
class Logger:
def log_message(self, message: str):
print("New logger: ", message)
# Adaptee (Legacy System)
class LegacyLogger:
def log_xml_message(self, xml_message: str):
print(f"Legacy Logger: Logging XML message - {xml_message}")
# Adapter
class XmlToJsonAdapter():
def __init__(self, legacy_logger: LegacyLogger):
self.legacy_logger = legacy_logger
def log_message(self, message: str):
# Convert the message from JSON to XML format
xml_message = self._convert_to_xml(message)
# Call the legacy logger with the adapted message
self.legacy_logger.log_xml_message(xml_message)
def _convert_to_xml(self, json_message: str) -> str:
# Simplified conversion for the sake of the example
# In a real scenario, you would have a proper JSON to XML conversion logic
return f"<xml>{json_message}</xml>"
# Client Code
def main():
# Legacy system
legacy_logger = LegacyLogger()
# Adapter to make the legacy system compatible with the new library
adapter = XmlToJsonAdapter(legacy_logger)
# New logging library expecting JSON messages
json_logger = Logger()
# Client code can now use the Logger interface
messages = ["Message 1", "Message 2", "Message 3"]
for message in messages:
# Legacy system is seamlessly integrated into the new library
adapter.log_message(message)
# New library can also be used directly
json_logger.log_message(message)
if __name__ == "__main__":
main()
Output
Legacy Logger: Logging XML message - <xml>Message 1</xml>
New logger: Message 1
Legacy Logger: Logging XML message - <xml>Message 2</xml>
New logger: Message 2
Legacy Logger: Logging XML message - <xml>Message 3</xml>
New logger: Message 3
Real-World Analogy
The power plug and socket standards are different in different countries. That’s why your Indian plug won’t fit a US socket. The problem can be solved by using a power plug adapter that has an Indian-style socket and a US-style plug.
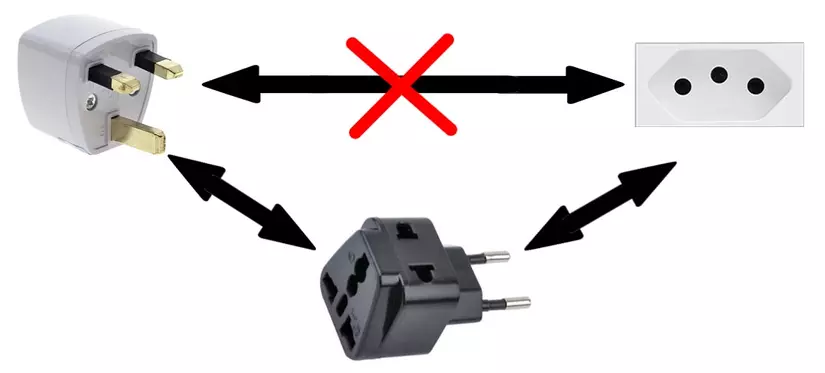
Conclusion
In conclusion, the Adapter Design Pattern serves as a cornerstone in software development, offering an elegant solution to the challenges of system interoperability. As technologies evolve and applications progress, the adaptability provided by this pattern becomes increasingly invaluable. By seamlessly integrating new components and ensuring compatibility between existing ones, the Adapter Design Pattern exemplifies the essence of a flexible and forward-looking approach to building robust software systems. Its significance underscores the broader importance of design patterns in fostering modularity, maintainability, and overall system resilience. As we navigate the ever-changing landscape of technology, the Adapter Design Pattern remains a reliable ally in achieving harmonious coexistence between diverse software components.
Resources
For further exploration, make sure to check out these helpful resources: