The SOLID principles are a set of five design principles that help software developers create more maintainable, understandable, and scalable code.
Let’s delve into each SOLID principle and explore how they contribute to building high-quality software.
Table of Contents
Solid Overview
Each principle can be applied at different levels of software design, including classes, methods, and modules. The explanation will mention classes for abbreviation but the same concept applies to the others.
S – Single Responsibility Principle: Every class should have only one responsibility, i.e., one reason to change.
O – Open/Closed Principle: Software entities should be easily extended with new functionality without altering existing code.
L – Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of its subclasses without affecting the “behaviour” of the program.
I – Interface Segregation Principle: Clients should not be forced to depend on interfaces they don’t use. Instead of creating large interfaces that serve multiple clients, it’s better to create smaller, more specific interfaces tailored to each client’s needs.
D – Dependency Inversion Principle: High-level modules should not depend on low-level modules; both should depend on abstractions.
These principles serve as a set of guidelines for writing clean, understandable, and flexible software.
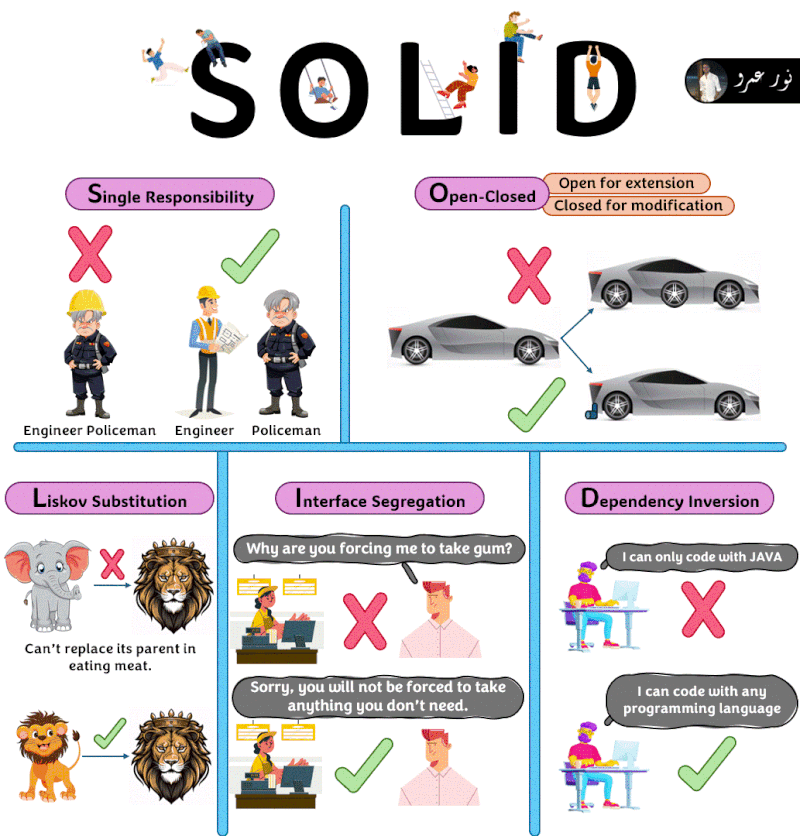
Single Responsibility Principle (SRP):
What?
A class should have only one reason to change, meaning it should have only one job or responsibility. This principle encourages keeping classes focused and avoids making them too complex.
Why?
SRP is essential for several reasons:
- Maintainability: Classes with a single responsibility are easier to maintain because changes related to that responsibility are isolated.
- Readability: Code becomes more readable as each class is focused on a specific task, making it easier for developers to understand and work with.
- Testability: Classes following SRP are more testable since they have clear boundaries and responsibilities, allowing for targeted unit testing.
How to use it?
To apply SRP effectively:
- Identify Responsibilities: Define clear responsibilities for each class based on its purpose and functionality.
- Separate Concerns: Ensure that each class is responsible for a single concern and delegates other tasks to appropriate classes or modules.
- Refactor as Needed: If a class starts to have multiple responsibilities, consider refactoring it into smaller, more focused classes that adhere strictly to SRP.
Example
Bad example
class UserManager:
def authenticate(self, username, password):
# Authentication logic
pass
def updateUserProfile(self, user_id, new_data):
# Update user profile logic
pass
In this example, the UserManager
class violates SRP by handling both user authentication and user profile management.
Good example
To apply SRP, we split these responsibilities into separate classes: AuthenticationService
and UserProfileService
.
class AuthenticatoinService:
def authenticate(self, username, password):
# Authentication logic
pass
class UserProfileSerivce:
def updateUserProfile(self, user_id, new_data):
# Update user profile logic
pass
Open/Closed Principle (OCP)
What?
Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means you should be able to add new functionality to a system without altering existing code. This is often achieved using techniques like inheritance, composition, and interfaces.
Why?
OCP is important for several reasons:
- Maintainability: By preventing direct modification of existing code, OCP helps maintain code stability and reduces the risk of introducing bugs.
- Scalability: OCP promotes extensibility, allowing new features to be added without altering existing code, thus making the system more scalable and adaptable to change.
- Reusability: Code that follows OCP is more reusable since new functionality can be added through extensions without affecting the core functionality.
Example
Example: Bad
class Shape:
def __init__(self, type):
self.type = type
def calculateArea(self):
if self.type == "circle":
# Circle area calculation logic
pass
elif self.type == "square":
# Square area calculation logic
pass
else:
raise ValueError("Unsupported shape type")
In this example, the Shape
class violates the Open/Closed Principle by directly checking for different types of shapes (circle
and square
) and implementing specific area calculation logic based on these types. If a new shape, such as a triangle, is introduced, the calculateArea
method needs to be modified, leading to the class being open for modification.
Example: Good
class Shape:
def calculateArea(self):
raise NotImplementedError("Subclasses must implement this method")
class Circle(Shape):
def calculateArea(self):
# Circle area calculation logic
pass
class Square(Shape):
def calculateArea(self):
# Square area calculation logic
pass
In this improved version, the Shape
class defines an abstract method calculateArea
that must be implemented by its subclasses (Circle
and Square
). Each subclass provides its own implementation of calculateArea
without modifying the base Shape
class. This adheres to the Open/Closed Principle, as the Shape
class is open for extension (by adding new shapes as subclasses) but closed for modification (existing code in Shape
doesn’t need to change when new shapes are added).
Resources
- https://codeburst.io/introduction-a1ba1f72b13
Liskov Substitution Principle (LSP)
What?
- Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- In other words, if S is a subtype of T, then objects of type T can be replaced with objects of type S without altering the desired behaviour of the program.
- It emphasises that derived components, which inherit from a base component, should be able to replace the base component in any scenario without causing unexpected errors or breaking the expected behaviour.
- This means that the derived components should support the same props, methods, and expected behaviour as the base component, while potentially adding additional features specific to their context, such as custom input validation or specific form submission logic. They should be substitutable for the base component without introducing errors or unexpected behaviour.
Why?
LSP is essential for several reasons:
- Interchangeability: Ensures that objects can be replaced with their subtypes without altering the program’s functionality, promoting code reusability and flexibility.
- Consistency: Maintains consistent behaviour across different classes in an inheritance hierarchy, leading to predictable and understandable code.
- Scalability: Facilitates the addition of new subclasses without impacting existing code, making the system more scalable and adaptable.
- Reusability
- Modularity
- Maintainability
Understanding LSP with a Use Case
- Imagine working on an e-commerce website with a product listing feature. You have a base Product component that renders the basic information of a product, such as its name, price, and image.
- Now, you need to introduce a new requirement to display additional details for a specific product type, let’s say “FeaturedProduct.” The featured products have extra information, such as a special badge and a promotional message.
- According to LSP, you should be able to create a derived component called FeaturedProduct that inherits from the base Product component and includes the additional features without breaking the expected behaviour.
- The FeaturedProduct component extends the base Product component by adding the special badge and promotional message rendering. It utilises the same props and methods expected from the base component while introducing the specific enhancements.
By adhering to LSP, you ensure that the FeaturedProduct component can be seamlessly used as a substitute for the Product component wherever product listings are rendered. This means that any part of the application expecting a Product component can safely receive a FeaturedProduct component without causing errors or unintended behaviour.
The benefit of applying LSP in this use case is that the code remains flexible and modular. The derived component extends the base component’s functionality while still adhering to the same contract, making it easy to introduce new types of products without modifying the existing codebase extensively.
Additionally, LSP allows for code reusability and maintainability. Other parts of the application that rely on the base Product component can be reused with the FeaturedProduct component without the need for significant changes. This promotes scalability and reduces development effort.
By designing and utilising components that follow LSP, you create a more robust and flexible codebase. The derived components can seamlessly replace their base components while providing additional features, enabling extensibility and maintaining consistency throughout the application.
Common Misconceptions
- Misconception: LSP is only about inheritance: One common misconception is that LSP is limited to inheritance-based relationships between components. While inheritance is one way to establish relationships between components, LSP is about substitutability and applies to any type of polymorphic relationship, including interfaces and composition.
- Misconception: LSP requires identical behaviour: Another misconception is that derived components must have identical behaviour to the base component. In reality, LSP allows for extended behaviour in derived components as long as it does not violate the contract and assumptions of the base component.
- Misconception: LSP requires all subcomponents to be used interchangeably: LSP does not imply that all subcomponents should be completely interchangeable with their base component in every context. It focuses on ensuring that derived components can be substituted for their base component when used in a context that expects the base component. Context-specific behaviour in derived components can be acceptable as long as it does not violate the LSP contract.
- Misconception: LSP eliminates the need for specialisation: Some may mistakenly believe that LSP discourages specialisation or customisation in derived components. However, LSP encourages the extension and customisation of behaviour as long as it adheres to the base component’s contract and does not break substitutability.
- Misconception: LSP is a strict rule: LSP should be considered as a guideline rather than a strict rule. While striving for LSP compliance is beneficial, there may be cases where violating LSP is justified to address specific design constraints or optimise performance. It’s essential to assess the trade-offs and consider the practical implications in such situations.
Understanding and dispelling these misconceptions helps developers apply LSP effectively in their codebase. It allows for the creation of robust and maintainable software systems by promoting substitutability, flexibility, and modularity while accounting for specific design considerations and requirements.
Example
class Product:
def __init__(self, name, price, image):
self.name = name
self.price = price
self.image = image
def display_info(self):
return f"Name: {self.name}, Price: ${self.price}"
class FeaturedProduct(Product):
def __init__(self, name, price, image, badge, promo_message):
super().__init__(name, price, image)
self.badge = badge
self.promo_message = promo_message
def display_info(self):
base_info = super().display_info()
return f"{base_info}, Badge: {self.badge}, Promo: {self.promo_message}"
# Usage example
product1 = Product("Product 1", 10, "product1.jpg")
product2 = FeaturedProduct("Product 2", 20, "product2.jpg", "Featured", "Limited Time Offer")
print(product1.display_info()) # Output: Name: Product 1, Price: $10
print(product2.display_info()) # Output: Name: Product 2, Price: $20, Badge: Featured, Promo: Limited Time Offer
Resource
Interface Segregation Principle (ISP)
What?
- Clients should not be forced to depend on interfaces they do not use.
- This principle advocates for creating smaller, specific interfaces rather than large, general-purpose ones. It helps avoid bloated interfaces and reduces the impact of changes on clients.
Why?
ISP is important for several reasons:
- Reduced Dependency: By defining smaller interfaces, ISP reduces the dependency of clients on unnecessary functionalities, leading to cleaner and more maintainable code.
- Better Encapsulation: Smaller interfaces encapsulate specific functionalities, making it easier to understand and reason about the codebase.
- Improved Testability: Code that follows ISP is easier to test as interfaces are focused on specific behaviours, allowing for targeted testing of individual components.
Example
from abc import ABC, abstractmethod
class Printable(ABC):
@abstractmethod
def print_document(self, document):
pass
class Scannable(ABC):
@abstractmethod
def scan_document(self):
pass
class Faxable(ABC):
@abstractmethod
def send_fax(self, number, document):
pass
class Copyable(ABC):
@abstractmethod
def copy_document(self):
pass
class InkjetPrinter(Printable, Scannable, Copyable):
def print_document(self, document):
print("Printing document:", document)
def scan_document(self):
print("Scanning document")
def copy_document(self):
print("Copying document")
class LaserPrinter(Printable, Copyable):
def print_document(self, document):
print("Printing document:", document)
def copy_document(self):
print("Copying document")
# Usage
inkjet = InkjetPrinter()
laser = LaserPrinter()
inkjet.print_document("Invoice")
inkjet.scan_document()
inkjet.copy_document()
laser.print_document("Report")
laser.copy_document()
Resource
- https://www.linkedin.com/pulse/interface-segregation-principle-isp-prithveesh-goel/
Dependency Inversion Principle (DIP)
What?
- High-level modules should not depend on low-level modules. Both should depend on abstractions (e.g., interfaces).
- Additionally, abstractions should not depend on details; details should depend on abstractions. This principle promotes decoupling and flexibility in software design.
But let’s break this down:
- Client: Your main class/code that runs the high-level module.
- High-Level Modules: Interface/Abstraction that your client uses.
- Low-Level Modules: Details of your interfaces/abstraction.
What it says that imagine you have a car and your different components are:
- Client: You as the person driving the car.
- High-Level Modules: The steering wheel and the gas/brake peddles.
- Low-Level Modules: Engine
Why?
DIP is important for several reasons:
- Reduced Coupling: By depending on abstractions rather than concrete implementations, DIP reduces coupling between modules, making the codebase more flexible and easier to maintain.
- Enhanced Testability: Code that follows DIP is easier to test as dependencies can be mocked or replaced with alternate implementations during testing, leading to more reliable and efficient testing.
- Improved Extensibility: DIP promotes a design where new implementations can be added or existing ones can be replaced without affecting the high-level modules that depend on them, thus improving extensibility and scalability.
Example
To apply DIP, define abstractions and have high-level modules depend on these abstractions rather than concrete implementations. Here’s an example in Python:
from abc import ABC, abstractmethod
class PaymentGateway(ABC):
@abstractmethod
def process_payment(self, amount):
pass
class PayPalGateway(PaymentGateway):
def process_payment(self, amount):
print(f"Processing payment of ${amount} via PayPal")
class StripeGateway(PaymentGateway):
def process_payment(self, amount):
print(f"Processing payment of ${amount} via Stripe")
class PaymentProcessor:
def __init__(self, gateway: PaymentGateway):
self.gateway = gateway
def process_payment(self, amount):
self.gateway.process_payment(amount)
# Usage
paypal_gateway = PayPalGateway()
stripe_gateway = StripeGateway()
payment_processor_paypal = PaymentProcessor(paypal_gateway)
payment_processor_stripe = PaymentProcessor(stripe_gateway)
payment_processor_paypal.process_payment(100)
payment_processor_stripe.process_payment(200)
Must Read Dependency Dynamics: Unveiling DIP, DI, and IoC
Conclusion
By incorporating the SOLID principles into your development practices with practical code examples, you can create codebases that are easier to understand, maintain, and extend. These principles foster modularity, flexibility, and scalability, leading to more robust and adaptable software systems. Remember, applying SOLID principles is not just about writing code; it’s about fostering a mindset of clean design and continuous improvement in software development.
1 thought on “Mastering the SOLID Principles: A Developer’s Guide”