Cohesion and coupling are two fundamental concepts in software engineering that describe the quality of the relationships between different components or modules within a software system. They are used to assess the design and maintainability of software. Let’s explore each concept:
Table of Contents
Understanding Cohesion
What Is Cohesion?
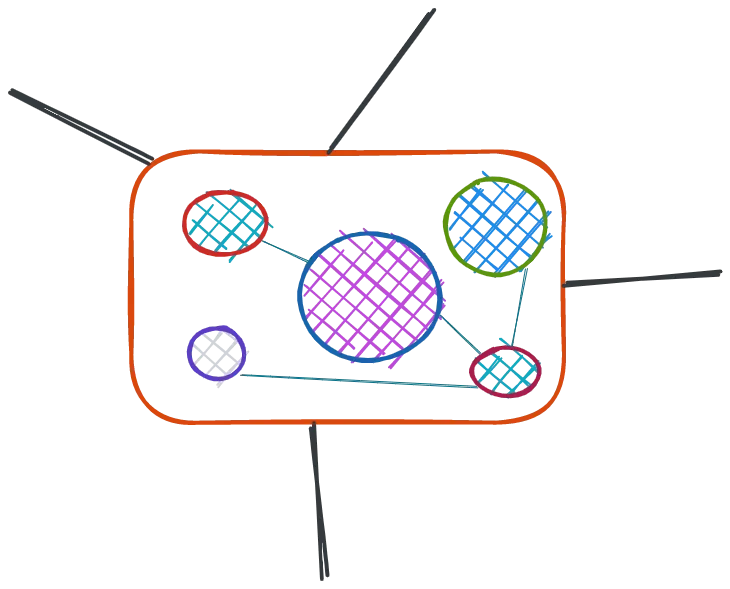
It refers to the degree to which the elements within a software module (such as functions, classes, or methods) are related to each other and work together to perform a single, well-defined task or responsibility.
In simpler terms, cohesion measures how closely the parts of a module are tied to a common purpose. A highly cohesive module will have elements that are strongly related to each other and collaborate to achieve a specific, focused objective.
Importance of High Cohesion
High cohesion is a fundamental design principle that offers numerous benefits to the development and maintenance of software systems. Here’s why cohesion is important:
- Maintainability: High cohesion makes code easier to maintain. When a module has a single, well-defined purpose, changes and updates to that module are localized and predictable. This reduces the risk of unintended side effects and makes it simpler to address issues or add new features.
- Readability and Understandability: Code with high cohesion is more readable and understandable. When all elements within a module are related to the same task or function, it’s easier for developers to comprehend the module’s purpose and how it fits into the larger system. This aids in code reviews, collaboration among team members, and troubleshooting.
- Testing and Debugging: Modules with high cohesion are easier to test and debug. Testing can focus on the specific functionality within a module, and debugging is more straightforward because the scope of potential issues is limited to the module itself. This reduces the complexity of the testing and debugging process.
- Reduced Risk: High cohesion reduces the risk of introducing unintended side effects or errors when making changes to the code. Changes are isolated to the module with the related responsibility, decreasing the likelihood of breaking other parts of the system. This is particularly important in mission-critical or safety-critical systems.
- Scalability: As a software system evolves, new features and enhancements can often be implemented as new cohesive modules. This modularity makes it easier to scale and extend the system without disrupting existing functionality. It promotes a structured approach to accommodating changing requirements.
- Documentation and Maintenance: Highly cohesive modules come with more natural and intuitive documentation because their purpose is clear and focused. This aids in maintaining the software over time, especially when new team members need to understand and work on the codebase.
- Parallel Development: In team-based development, high cohesion allows different team members to work on different modules concurrently with minimal conflicts. This can improve development speed and efficiency.
Types of Cohesion
There are several types or levels of cohesion that describe the different ways in which elements within a module can be related to each other. These types of cohesion are arranged from the highest level of cohesion to the lowest:
Functional Cohesion: This is the highest level of cohesion, and it represents the ideal state. In a functionally cohesive module, all elements work together to perform a single, well-defined task or function.
Example: A module that calculates the square root of a number, where all elements are related to the calculation of the square root.
Sequential Cohesion: In this type of cohesion, elements are related because they are executed sequentially. While not as ideal as functional cohesion, elements in a sequentially cohesive module are still related because they are part of a common sequence of execution.
Example: A module that reads data from a file, processes it, and then writes the results to another file.
Temporal Cohesion: Elements in a module have temporal cohesion when they are grouped together because they are executed at the same time, but not necessarily for the same purpose. This type of cohesion is weaker than sequential cohesion.
Example: A module that initializes various system resources during system startup.
Communicational Cohesion: In a module with communicational cohesion, elements are grouped together because they operate on the same data or share data between each other. While they may not perform a single function, they are related through data communication.
Example: A module that processes user input data, validates it, and then sends it to a database module for storage.
Procedural Cohesion:Procedural cohesion occurs when elements are grouped together because they contribute to a common procedure or process, but the relationship may not be as strong as in functional cohesion.
Example: A module that handles various steps in a document creation process, such as formatting, spell-checking, and printing.
6. Coincidental Cohesion: Coincidental cohesion is the lowest level of cohesion and represents a weak or arbitrary relationship among elements within a module. Elements are grouped together without a meaningful or logical connection.
Example: A utility module that contains unrelated functions for different purposes, such as mathematical calculations and file manipulation.
In software design, the goal is to achieve high cohesion, particularly functional cohesion, while avoiding or minimizing lower levels of cohesion. High cohesion leads to more maintainable and understandable code because the elements within a module have a clear and well-defined purpose, making it easier to reason about and work with the code.
Design Guidelines for Achieving High Cohesion
Achieving high cohesion in software design is a crucial goal because it leads to more maintainable, readable, and robust code. Here are some design guidelines and best practices for achieving high cohesion in your software modules:
- Single Responsibility Principle (SRP): Follow the SRP from the SOLID principles. Each module or class should have only one reason to change. If a module has multiple responsibilities, consider breaking it into smaller, more focused modules.
- Separation of Concerns: Separate different concerns or responsibilities into distinct modules or classes. For example, separate user interface logic from business logic and data access.
- Modular Design: Break your software into small, self-contained modules that have specific, well-defined tasks. Each module should encapsulate a single piece of functionality and perform it well.
- Encapsulation: Use encapsulation to hide the internal details of a module. This reduces the dependencies between modules and ensures that each module’s behaviour can be understood and modified independently.
- Information Hiding: Limit the visibility of module internals to the outside world. Expose only the necessary interfaces and hide implementation details. This promotes loose coupling and high cohesion.
- Use of Interfaces and Abstraction: Create clear and well-defined interfaces for your modules. Encourage the use of abstractions to define contracts and interactions between modules, making it easier to replace or extend them.
- Keep Methods and Functions Focused: Ensure that methods and functions within a module have a single, well-defined purpose. Avoid creating large methods that perform multiple tasks. If necessary, break down complex methods into smaller, reusable submethods.
- Avoid Global Variables and State: Minimize the use of global variables and shared state between modules. Instead, pass data as parameters or use dependency injection to provide necessary information.
- Adhere to Design Patterns: Utilize design patterns like the Factory Pattern, Strategy Pattern, and Observer Pattern to promote high cohesion. These patterns encourage modular, loosely coupled designs.
- Regular Code Reviews: Conduct regular code reviews to identify and address cohesion issues. Code reviews provide an opportunity to assess whether modules are adhering to the design principles and maintaining high cohesion.
- Refactor When Necessary: When you encounter modules with low cohesion, be prepared to refactor your code. Breaking apart large, monolithic modules into smaller, more cohesive ones can improve the overall design.
- Documentation: Document the purpose and responsibilities of each module and its public interface. Good documentation helps developers understand the module’s role within the system.
- Test-Driven Development (TDD): Adopt TDD practices to create tests for your modules as you develop them. This ensures that each module has a well-defined and testable behaviour, reinforcing high cohesion.
Examples of High Cohesion
Math Library Functions:
- Module/Class: A math library module or class.
- Purpose: Contains functions for mathematical operations like addition, subtraction, multiplication, and division.
- Explanation: All the functions within this module are related to mathematical operations, and they work together to perform these operations. This is an example of functional cohesion.
File Handling Module:
- Module/Class: A file handling module.
- Purpose: Provides functions for reading, writing, and manipulating files.
- Explanation: All the functions in this module are related to file I/O and file manipulation. They work together to handle files, demonstrating functional cohesion.
User Authentication Class:
- Class: A user authentication class in a web application.
- Purpose: Manages user authentication and authorization.
- Explanation: This class contains methods for user login, user logout, and checking user permissions. All these methods are related to user authentication, making this class an example of functional cohesion.
Sorting Algorithms Package:
- Package/Module: A collection of sorting algorithms.
- Purpose: Provides various sorting algorithms such as bubble sort, quicksort, and merge sort.
- Explanation: Each sorting algorithm within the package has a specific sorting task, and they are encapsulated within the same package. This demonstrates functional cohesion within the package.
Graphics Library:
- Library: A graphics library for rendering 2D shapes.
- Purpose: Contains classes and functions for drawing shapes, lines, and text on a canvas.
- Explanation: All the classes and functions in this graphics library are focused on rendering graphics. They work together to provide graphic-related functionality, demonstrating functional cohesion.
Database Access Module
- Module/Class: A module for database access.
- Purpose: Provides functions for connecting to a database, executing SQL queries, and handling database transactions.
- Explanation: All the functions within this module are related to database access and manipulation. They collaborate to interact with a database, showing functional cohesion.
Billing Module in an E-commerce System:
- Module/Class: A billing module in an e-commerce application.
- Purpose: Calculates and generates invoices for customer orders.
- Explanation: This module contains functions that handle all aspects of billing, including calculating prices, applying discounts, and generating invoices. All functions are tightly related to the billing process, representing functional cohesion.
In each of these examples, you can see that the modules, classes, or functions have a well-defined and focused purpose. They encapsulate related functionality, making the code more understandable, maintainable, and modular. This adherence to high cohesion principles helps create software that is easier to work with and less prone to errors.
Understanding Coupling
What Is Coupling?
Coupling refers to the degree of interdependence or connection between different modules, classes, components, or subsystems within a software system. It assesses how closely one part of the system relies on or interacts with another part. Coupling can be classified into different levels or types, which describe the strength of the relationships between these parts.
Low coupling is generally considered a desirable design characteristic, as it promotes modularity, maintainability, and flexibility in software systems. Reducing coupling means that changes to one part of the system have minimal impact on other parts, making it easier to develop, test, and maintain the software.
Conversely, high coupling implies a strong relationship between components, which can lead to challenges when modifying or extending the software because changes in one part of the system may necessitate changes in other closely coupled parts. High coupling can result in less modular and less flexible code.
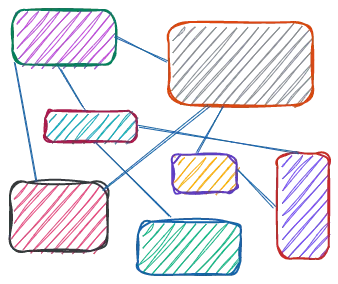
Importance of Low Coupling:
- Maintainability and Flexibility: Changes in one part of the system have minimal impact on other parts, making it easier to maintain and modify.
- Code Reusability: Modular and loosely coupled systems are more reusable, as components can be used in different contexts without significant modification.
- Parallel Development: Different modules can be developed independently by different teams or developers, speeding up the development process.
- Scalability: Low coupling allows for easier scaling of the system, as new features or components can be added without disrupting existing functionality.
Types of Coupling:
Coupling can be categorized into different types, each representing a different level of interdependence between modules:
- Low Coupling: Desirable as it minimizes the dependencies between modules, making the system more modular and easier to maintain.
- Control Coupling: Occurs when one module controls the behaviour of another by passing information like flags or parameters. It’s better than other types but should be minimized.
- Data Coupling: Involves modules sharing data, but not each other’s internal logic. Modules are loosely connected through data.
- Stamp Coupling: Modules share a complex data structure or record. While better than data coupling, it still represents a form of dependency.
- Content Coupling: Represents the highest level of coupling, where modules depend on each other’s internal details, such as accessing variables or methods directly.
Design Guidelines for Achieving Low Coupling:
- Dependency Injection: Inject dependencies into a component rather than having the component create or find them. This promotes flexibility and testability.
- Interface-based Programming: Use interfaces to define contracts between components, allowing for loose coupling by focusing on the interface rather than the implementation.
- Loose Coupling Patterns: Utilize design patterns that promote loose coupling, such as the Observer Pattern or Strategy Pattern.
- Decoupling Layers: Design the architecture with distinct layers, each with well-defined responsibilities, to minimize dependencies between layers.
Examples of Low Coupling in points
- Modular Component Design: A system is designed with modular components, each responsible for a specific functionality (e.g., user authentication, data processing, and reporting). These modules communicate through well-defined interfaces, reducing interdependence.
- Dependency Injection: Instead of a component creating its dependencies, it receives them through dependency injection. For instance, a service may receive a database connection or a logger as parameters, promoting flexibility and testability.
- Interface-based Programming: An application uses interfaces to define contracts between different components. For instance, multiple data storage providers (e.g., databases, file systems) implement a common interface, allowing the application to switch between them easily.
- Loose Coupling Patterns: Applying the Observer Pattern, where an object (subject) maintains a list of its dependents (observers) that are notified of state changes. This allows for a flexible and decoupled way of handling events.
- Decoupling Layers: A three-tier architecture with clear separation of presentation, business logic, and data access layers. Each layer interacts with the layer below through well-defined interfaces, reducing dependencies and promoting maintainability.
- Event-Driven Architecture: Components communicate through events, where one component emits an event, and others respond to it. This allows for loosely coupled interactions between components, as they don’t need to know each other’s details.
- Microservices Architecture: A system is built as a collection of independent microservices, each responsible for a specific business capability. These microservices communicate through APIs, allowing them to evolve independently and reducing dependencies.
- Service-Oriented Architecture (SOA): A system is designed as a set of loosely coupled services that communicate through standardized interfaces (e.g., web services). Each service encapsulates specific functionality, promoting reusability and flexibility.
- Component-Based Development: Developing software using components that provide well-defined interfaces. These components can be easily replaced or upgraded without affecting the overall system, promoting flexibility and maintainability.
- Dependency Inversion Principle: Applying the Dependency Inversion Principle from SOLID, where high-level modules depend on abstractions, and low-level modules implement those abstractions. This allows changes in low-level modules without affecting high-level modules.
Conclusion
Cohesion and coupling serve as fundamental pillars in the realm of software design. Striving for high cohesion, where elements within a module work closely toward a singular purpose, enhances code readability, maintainability, and reusability. Simultaneously, advocating for low coupling, minimizing interdependencies between modules, allows for a more flexible, scalable, and resilient system. Balancing these principles empowers software architects to create modular, adaptable solutions, laying the groundwork for sustainable, high-quality software in an ever-evolving technological landscape.
Resources
For further exploration, make sure to check out these helpful resources