In the dynamic landscape of software development, the Template Method Design Pattern emerges as a strategic tool for crafting robust and flexible code. At its core, this pattern introduces a blueprint for algorithmic structures, allowing developers to define a standardized framework in an abstract class while enabling customization through subclasses. By providing a clear separation between generic and specific implementations, the Template Design Pattern fosters code reusability, consistency, and maintainability. In the upcoming exploration, we will unravel the simplicity and power of this design pattern, showcasing how it becomes a cornerstone for creating scalable and elegant software solutions.
Table of Contents
What?
The template method pattern is a behavioural design pattern that defines the outline or structure of an algorithm in a base class but delegates some steps of the algorithms to its base class
Why?
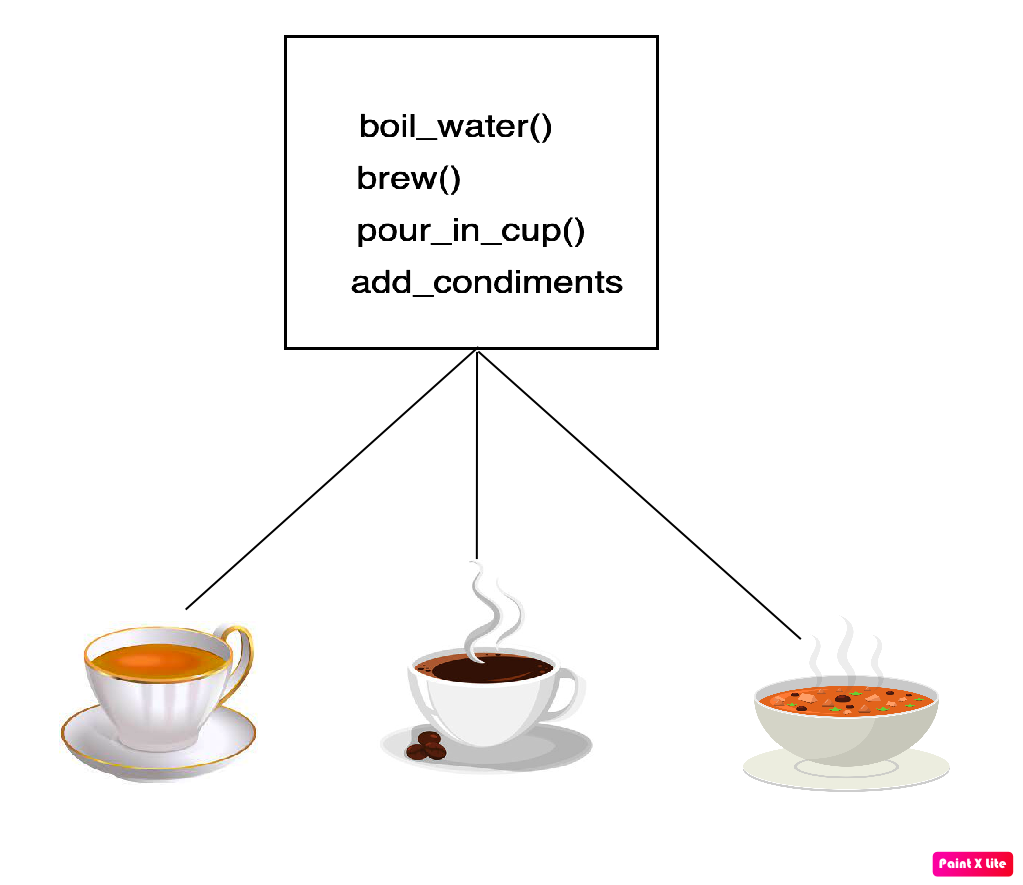
Let’s understand this through an example. Imagine you are developing code for a beverage vending machine that offers a variety of drinks such as coffee, tea, and soups. Initially, you write individual functions for each type of beverage, each containing the necessary steps for preparation.
Upon closer analysis of your code, you realize that the majority of the steps are identical across different beverage types. This redundancy poses a problem, as it leads to code duplication and makes the codebase less maintainable.
The Template Design Method comes into play to address this issue. It aims to solve the problem of repetitive code by providing a structured way to define a common algorithm while allowing specific steps to be customized in subclasses. In the context of the beverage vending machine, the template design pattern would enable you to create a template or blueprint for the overall beverage preparation process.
With this template, you can encapsulate the common steps, such as boiling water, brewing, pouring into a cup, and adding condiments, in an abstract class. Subclasses for each beverage type (coffee, tea, soup) can then inherit from this abstract class and provide their specific implementations for the steps that vary.
By doing so, the Template Design Method promotes code reusability, maintains a consistent structure across different beverages, and facilitates easier maintenance. Any changes or additions to the overall preparation process can be made in the abstract class, ensuring that modifications are centralized and applied uniformly to all beverage types.
In essence, the template design pattern streamlines the development process, making the codebase more modular, readable, and adaptable to future changes or additions to the beverage offerings in the vending machine.
Code
from abc import ABC, abstractmethod
# Step 1: Create an abstract class (Template)
class BeverageTemplate(ABC):
# template method
def prepare_beverage(self):
self.boil_water()
self.brew()
self.pour_in_cup()
self.add_condiments()
@abstractmethod
def boil_water(self):
pass
@abstractmethod
def brew(self):
pass
@abstractmethod
def pour_in_cup(self):
pass
@abstractmethod
def add_condiments(self):
pass
# Step 2: Create concrete classes (Subclasses) for specific beverages
class Tea(BeverageTemplate):
def boil_water(self):
print("Boiling water for tea")
def brew(self):
print("Brewing tea leaves")
def pour_in_cup(self):
print("Pouring tea into cup")
def add_condiments(self):
print("Adding lemon to tea")
class Coffee(BeverageTemplate):
def boil_water(self):
print("Boiling water for coffee")
def brew(self):
print("Brewing coffee grounds")
def pour_in_cup(self):
print("Pouring coffee into cup")
def add_condiments(self):
print("Adding sugar and milk to coffee")
# Step 3: Client code
if __name__ == "__main__":
print("Preparing tea:")
tea = Tea()
tea.prepare_beverage()
print("\nPreparing coffee:")
coffee = Coffee()
coffee.prepare_beverage()
Function prepare_beverage() is a template method
Use Case of the Template Method Design
- Game Characters: In games, you can have different characters, but their behavior is almost the same; they can walk, run, or fight.
- Document Editing: In a text processing application, such as a word processor, there is an option to export documents into various formats like PDF, Word files, or plain text.
- Sorting Algorithms: In sorting algorithms, different methods share common steps like comparing elements and swapping positions. Despite varying approaches, the fundamental actions, such as comparisons and swaps, remain consistent.
- Test Frameworks: When developing automated test frameworks, common steps include setting up test environments, executing tests, and reporting results. Different test scenarios, like unit tests or integration tests, can be implemented as subclasses while adhering to the overarching testing template.
- Product Assembly: In manufacturing processes, product assembly lines involve common steps like inspection, assembly, and packaging. While different products may have unique assembly requirements, the general assembly process can be standardized using the Template Design Pattern.
- Financial Modeling – Investment Strategies: In financial modeling, various investment strategies share common steps like data analysis, risk assessment, and decision-making. The Template Design Pattern can be applied to structure these strategies, with abstract classes defining general steps and subclasses implementing specific investment strategies.
These corrected examples highlight different scenarios where the Template Design Pattern can be applied to provide a consistent and customizable approach to common processes.
Conclusion
In conclusion, the Template Design Pattern emerges as a powerful and versatile tool in software development, offering a structured and reusable approach to address commonalities while accommodating variations in specific contexts. By defining an abstract template that outlines the skeleton of an algorithm, this pattern enables the creation of consistent and modular code structures. Its applications span various domains, from web frameworks and game development to manufacturing processes and financial modeling. The Template Design Pattern not only enhances code organization and maintainability but also promotes scalability and adaptability in the face of evolving requirements. Embracing this pattern empowers developers to streamline development processes, reduce redundancy, and create code that is both readable and extensible.
Resources
For further exploration, make sure to check out these helpful resources: